how to program alexa
# How to Program Alexa: A Comprehensive Guide
In the age of smart technology, voice-activated assistants like amazon ‘s Alexa have become an integral part of our daily lives. From controlling smart home devices to providing personalized responses, Alexa’s versatility is unmatched. If you’re interested in harnessing the power of Alexa for your projects or enhancing your own smart home experience, this guide will walk you through the steps of programming Alexa, covering everything from the basics to advanced skills development.
## Understanding Alexa and Its Capabilities
Before diving into programming, it’s essential to understand what Alexa is and what it can do. Alexa is a cloud-based voice service developed by Amazon that can perform a variety of tasks using voice commands. Some of its capabilities include:
1. **Smart Home Control**: Alexa can control various smart home devices, such as lights, thermostats, and security cameras.
2. **Information Retrieval**: It can answer questions, provide weather updates, and deliver news briefings.
3. **Music and Entertainment**: Alexa can play music, audiobooks, and podcasts, as well as control compatible entertainment systems.
4. **Reminders and Alarms**: Users can set timers, reminders, and alarms through voice commands.
5. **Third-Party Skills**: Developers can create custom skills that expand Alexa’s functionality.
Understanding these capabilities will help you identify how you can leverage Alexa for your programming projects.
## Setting Up Your Development Environment
To program Alexa, you’ll need to set up a development environment. Here’s how you can get started:
1. **Create an Amazon Developer Account**: Visit the [Amazon Developer Portal](https://developer.amazon.com/) and sign up for a free account. This account will allow you to access Alexa’s developer tools and resources.
2. **Familiarize Yourself with AWS**: Alexa is built on Amazon Web Services (AWS). Understanding the basics of AWS will be beneficial, especially if you plan to use AWS Lambda for your skills. Sign up for an AWS account if you don’t have one.
3. **Install Node.js**: Many Alexa skills are built using Node.js, a JavaScript runtime that allows you to run JavaScript on the server side. Download and install Node.js from the [official website](https://nodejs.org/).
4. **Select a Code Editor**: Choose a code editor that you are comfortable with. Popular options include Visual Studio Code, Atom, and Sublime Text. These editors offer features that enhance coding efficiency, such as syntax highlighting and debugging tools.
## Building Your First Alexa Skill
Now that your development environment is set up, it’s time to build your first Alexa skill. Follow these steps to create a simple skill that responds to user queries:
### Step 1: Define Your Skill
Before coding, define what your skill will do. For this example, let’s create a “Hello World” skill that responds with a friendly greeting.
### Step 2: Create a New Skill in the Developer Console
1. Log in to the [Alexa Developer Console](https://developer.amazon.com/alexa/console/ask).
2. Click on “Create Skill.”
3. Provide a name for your skill (e.g., “Hello World”).
4. Choose the custom model option and click “Create Skill.”
### Step 3: Configure the Skill
1. In the skill builder, navigate to the “Invocation” section and set a name that users will say to activate your skill (e.g., “hello world”).
2. Move to the “Intents” section and create a new intent called “HelloIntent.” This intent will handle the user’s request.
3. Add sample utterances that users might say to trigger this intent, such as “say hello,” “greet me,” or “hello world.”
### Step 4: Set Up the Backend
1. In the “Endpoint” section, select “AWS Lambda ARN” and choose a region (e.g., US East).
2. Go to the AWS Lambda console and create a new Lambda function. Choose “Author from scratch” and select Node.js as the runtime.
3. Write the function code that will handle the intent. Here’s a simple example code:
“`javascript
const Alexa = require(‘ask-sdk-core’);
const HelloIntentHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === ‘IntentRequest’
&& Alexa.getIntentName(handlerInput.requestEnvelope) === ‘HelloIntent’;
},
handle(handlerInput) {
const speakOutput = ‘Hello, world!’;
return handlerInput.responseBuilder
.speak(speakOutput)
.getResponse();
}
};
const LaunchRequestHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === ‘LaunchRequest’;
},
handle(handlerInput) {
const speakOutput = ‘Welcome to Hello World skill! You can say hello to me.’;
return handlerInput.responseBuilder
.speak(speakOutput)
.getResponse();
}
};
const HelpIntentHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === ‘IntentRequest’
&& Alexa.getIntentName(handlerInput.requestEnvelope) === ‘AMAZON.HelpIntent’;
},
handle(handlerInput) {
const speakOutput = ‘You can say hello to me!’;
return handlerInput.responseBuilder
.speak(speakOutput)
.getResponse();
}
};
const CancelAndStopIntentHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === ‘IntentRequest’
&& (Alexa.getIntentName(handlerInput.requestEnvelope) === ‘AMAZON.CancelIntent’
|| Alexa.getIntentName(handlerInput.requestEnvelope) === ‘AMAZON.StopIntent’);
},
handle(handlerInput) {
const speakOutput = ‘Goodbye!’;
return handlerInput.responseBuilder
.speak(speakOutput)
.getResponse();
}
};
const SessionEndedRequestHandler = {
canHandle(handlerInput) {
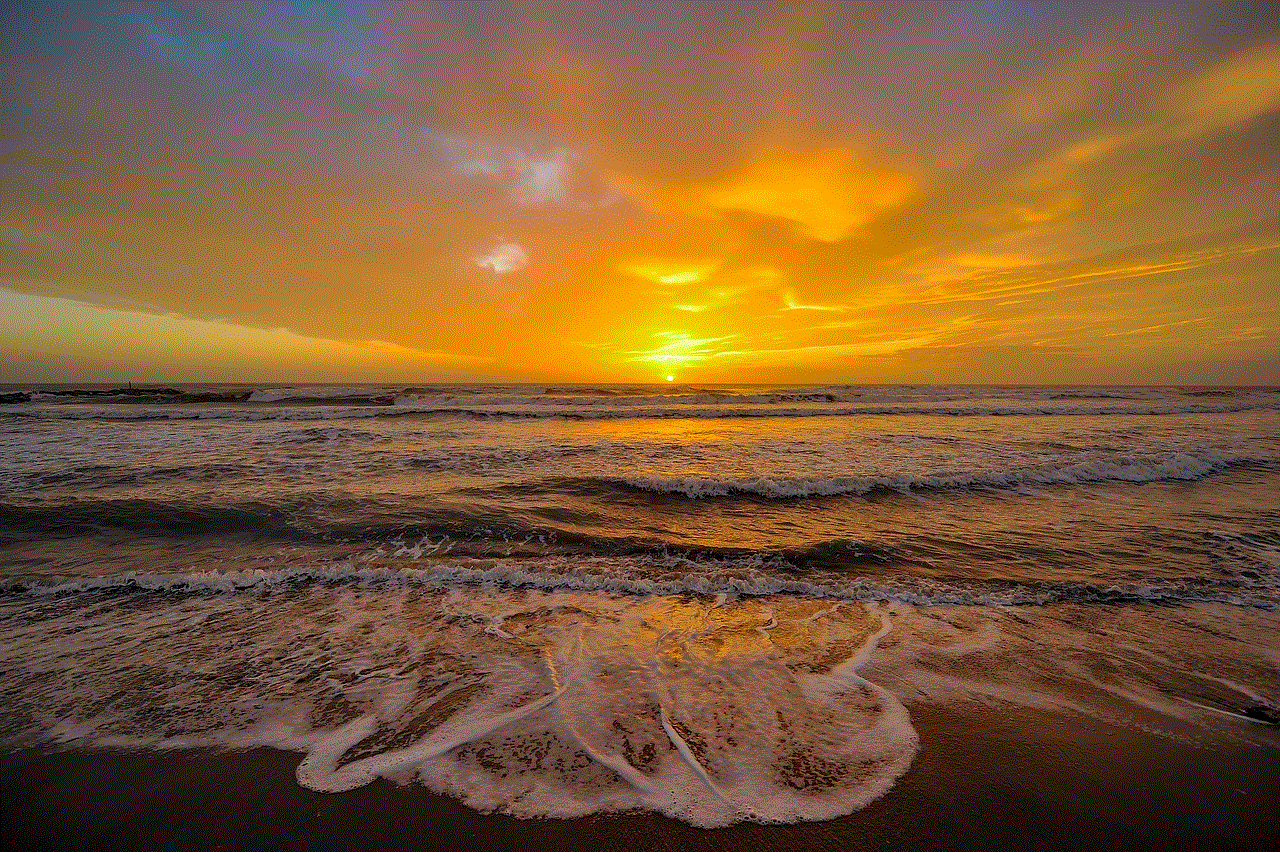
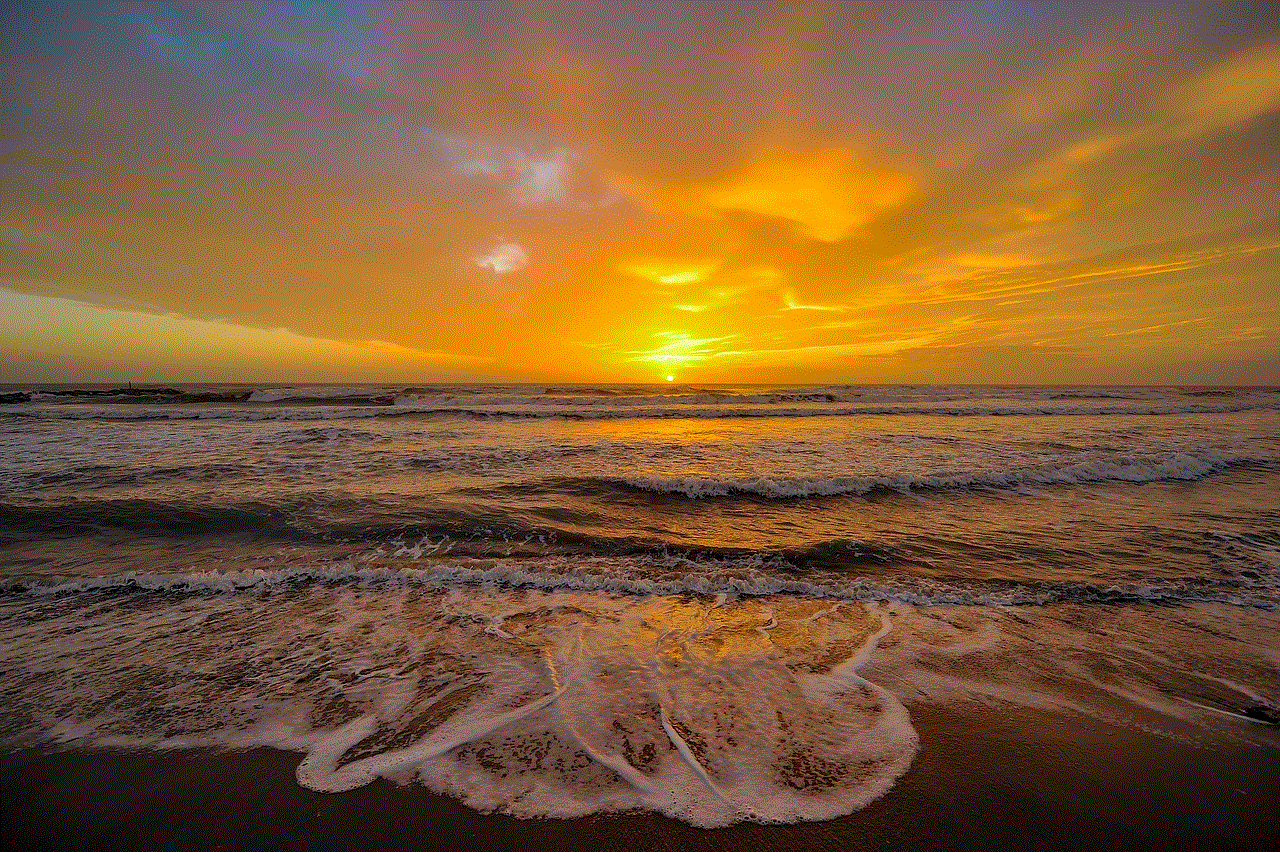
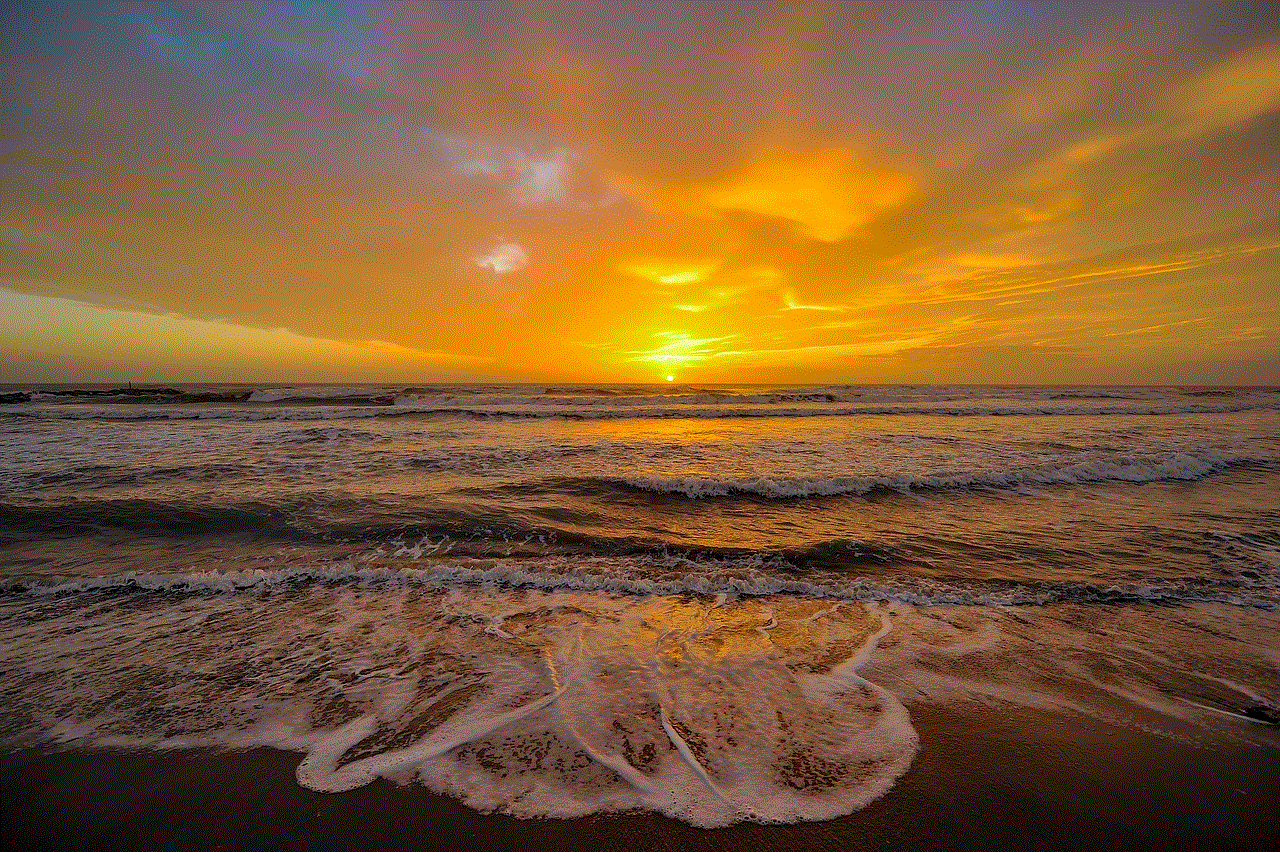
return Alexa.getRequestType(handlerInput.requestEnvelope) === ‘SessionEndedRequest’;
},
handle(handlerInput) {
return handlerInput.responseBuilder.getResponse();
}
};
const ErrorHandler = {
canHandle() {
return true;
},
handle(handlerInput, error) {
console.log(`Error handled: ${error.message}`);
const speakOutput = ‘Sorry, I had trouble doing what you asked. Please try again.’;
return handlerInput.responseBuilder
.speak(speakOutput)
.getResponse();
}
};
const skillBuilder = Alexa.SkillBuilders.custom();
exports.handler = skillBuilder
.addRequestHandlers(
LaunchRequestHandler,
HelloIntentHandler,
HelpIntentHandler,
CancelAndStopIntentHandler,
SessionEndedRequestHandler
)
.addErrorHandlers(ErrorHandler)
.lambda();
“`
### Step 5: Deploy Your Skill
1. Copy the ARN from your Lambda function and paste it into the endpoint section of your Alexa skill.
2. Save and build the model in the Alexa Developer Console.
3. Test your skill using the built-in simulator to ensure it responds correctly.
## Testing and Debugging Your Skill
Testing is a crucial part of skill development. Alexa provides a testing tool within the developer console that allows you to simulate voice interactions.
1. In the Alexa Developer Console, navigate to the “Test” tab.
2. Ensure that the test switch is set to “Development.”
3. Type or say phrases that invoke your skill (e.g., “Alexa, open hello world”).
4. Review the responses and make necessary adjustments to your code if something doesn’t work as intended.
Debugging is an essential skill in programming. Use logging within your Lambda function to monitor the flow of execution and catch any errors that may arise. You can view these logs in the AWS CloudWatch console.
## Enhancing Your Skill with Rich Features
Once you have a basic skill up and running, consider enhancing it with additional features. Here are some ideas to take your skill to the next level:
### 1. Adding Slots
Slots are variables that allow you to capture user input. For example, if you want to create a skill that provides personalized greetings, you could add a slot for the user’s name.
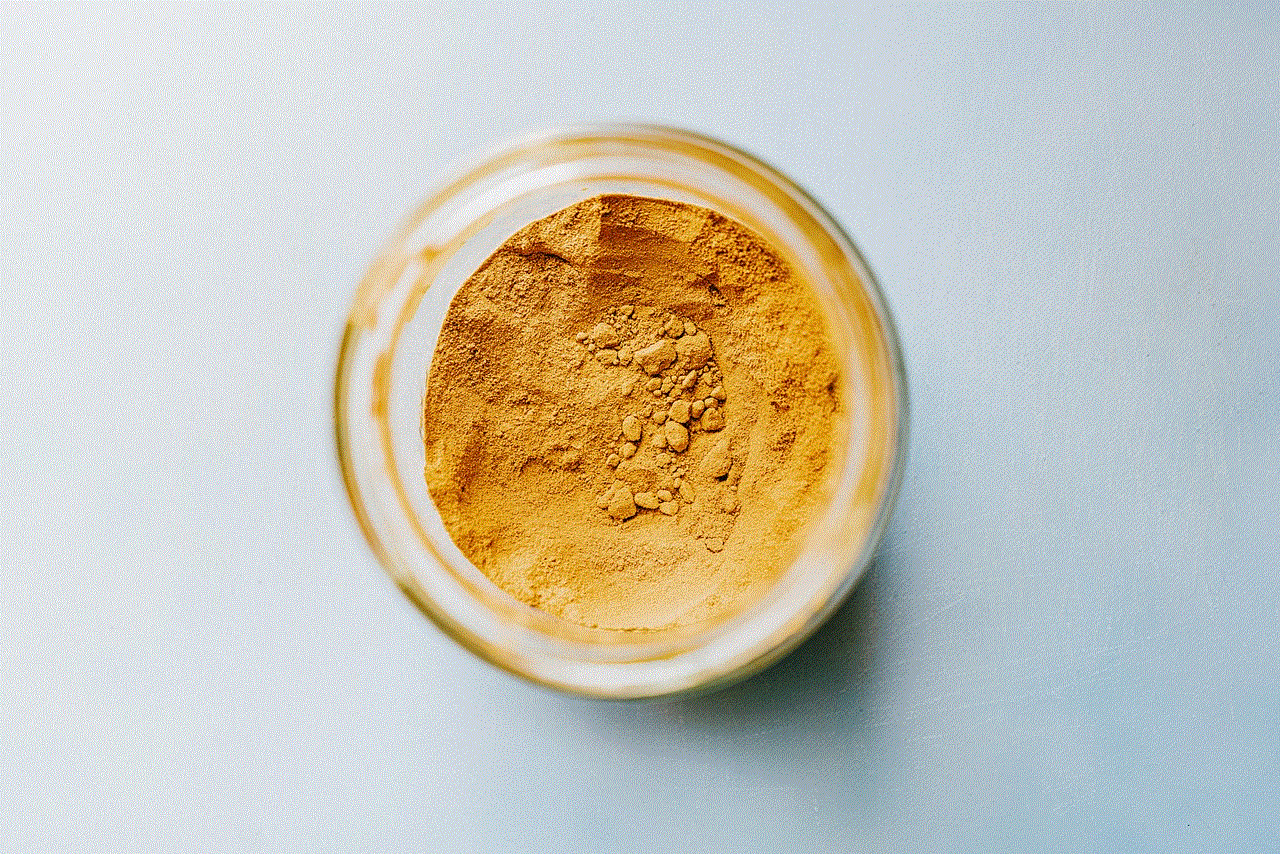
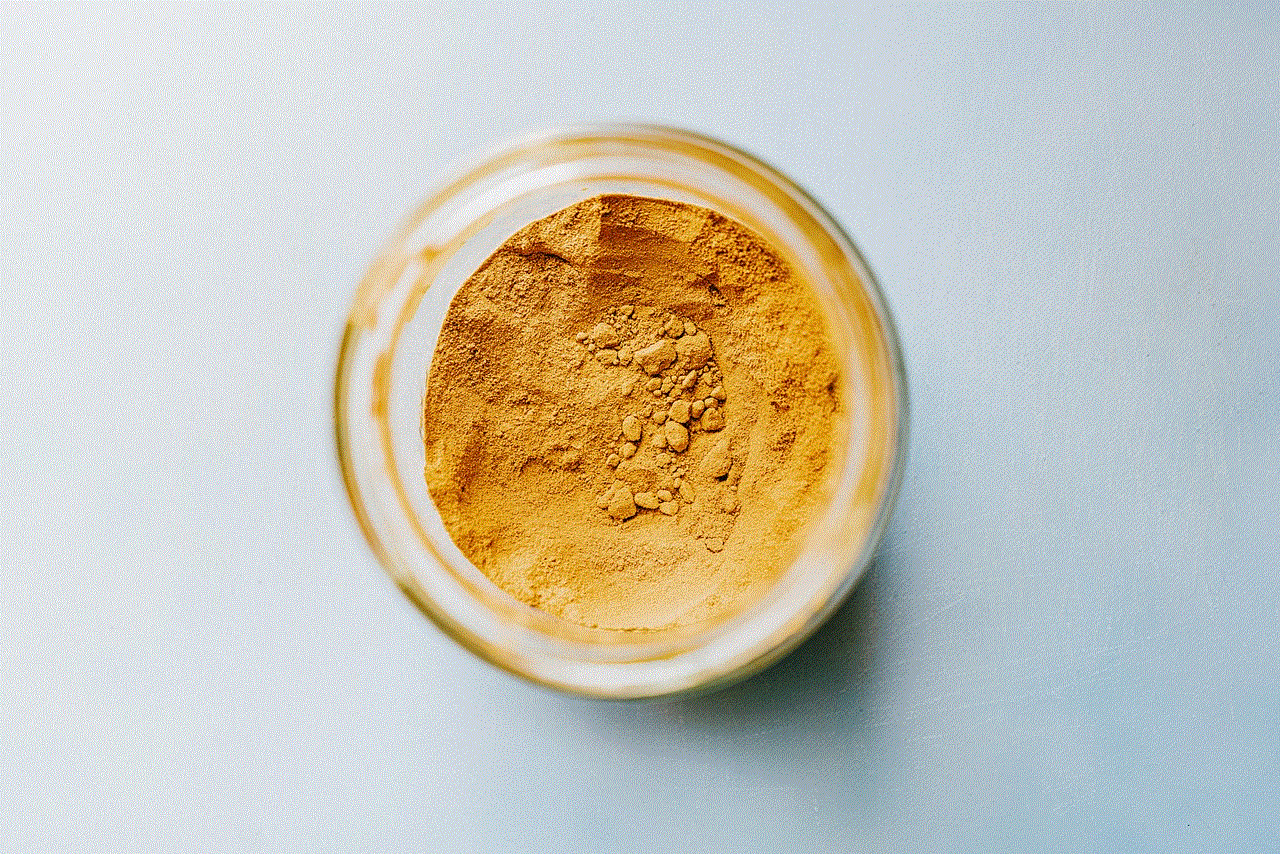
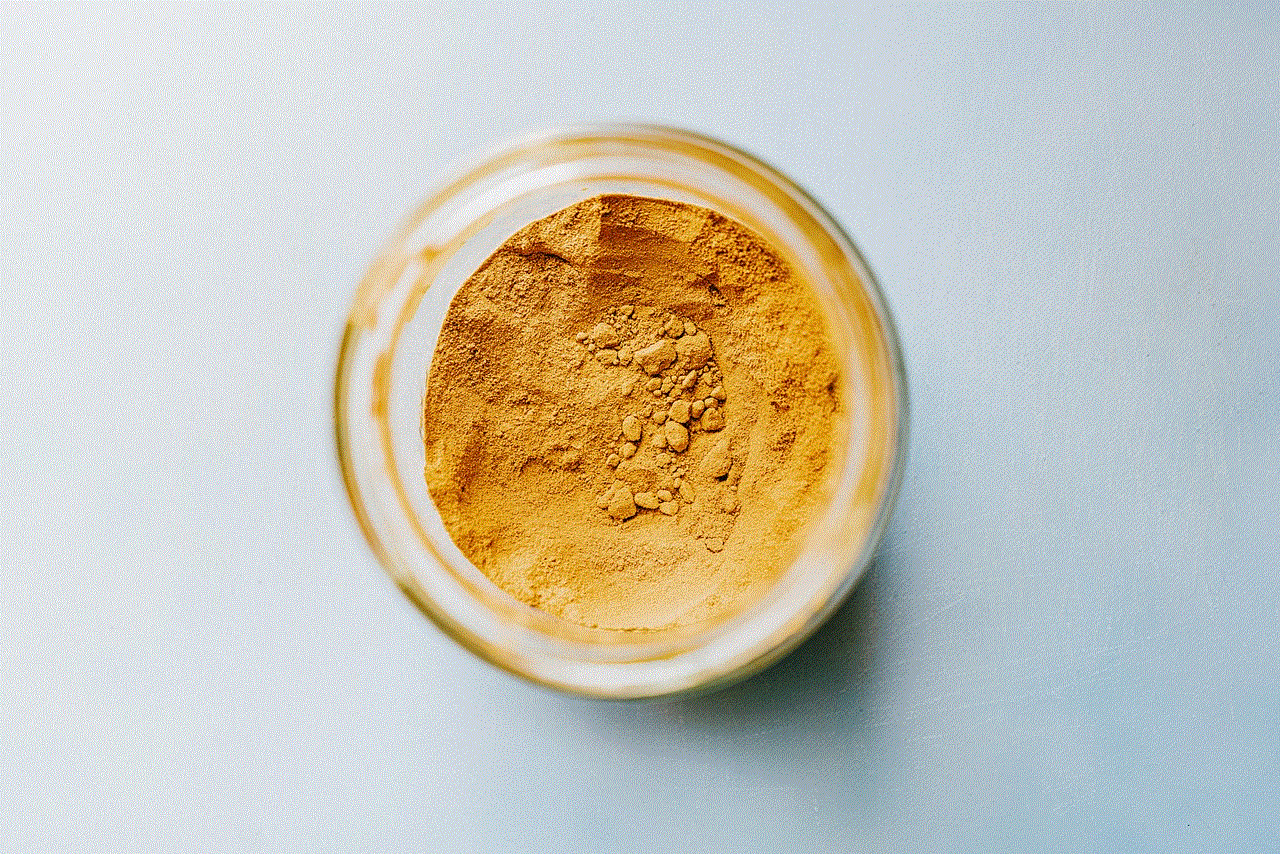
1. In the Alexa Developer Console, go to your HelloIntent.
2. Add a new slot called “Name” and specify the type as “AMAZON.Person” or create a custom slot type.
3. Update your intent to include the slot, and modify your code to use the captured name in the response.
### 2. Implementing Dialog Management
Dialog management allows for a more natural conversation flow. You can prompt users for additional information and ensure they provide the necessary details to fulfill their requests.
1. Define the dialog model in your intent by specifying required slots.
2. Use the `DialogDelegate` directive to manage the conversation and guide users through the necessary inputs.
### 3. Using APL for Visual Displays
If your skill is compatible with devices that have screens, consider using Alexa Presentation Language (APL) to provide visual responses. APL allows you to create rich visual interfaces for your skill.
1. Create an APL document that defines the layout and content for your skill’s visual response.
2. Integrate APL into your skill by using the `Alexa.Presentation.APL.RenderDocument` directive in your response.
### 4. Integrating APIs for Dynamic Responses
To make your skill more engaging, consider integrating external APIs to provide dynamic content. For instance, you could fetch live data, such as weather updates or news articles, and present them to the user.
1. Use the `axios` or `request` library to make HTTP requests to external APIs.
2. Process the response and format it for Alexa’s output.
## Publishing Your Skill
After testing and refining your skill, you may want to share it with the world. Publishing your skill involves several steps:
1. **Complete the Skill Information**: Navigate to the “Distribution” tab in the Alexa Developer Console. Fill out the skill information, including a detailed description, keywords, and icons.
2. **Set Up Privacy and Compliance**: Ensure your skill complies with Amazon’s policies. Provide information on how you handle user data, and if applicable, include a privacy policy URL.
3. **Submit for Certification**: Once you’re satisfied with your skill, submit it for certification. Amazon will review your skill to ensure it meets their guidelines. The review process may take a few days.
4. **Promote Your Skill**: After approval, promote your skill through social media, blogs, or other platforms to attract users. Encourage feedback to improve your skill over time.
## Advanced Programming Techniques
As you become more proficient in programming Alexa, consider exploring advanced techniques that can enhance your skills further.
### 1. Using Custom Interfaces
Alexa allows developers to create custom interfaces that extend the standard capabilities of the voice assistant. By utilizing the Alexa Skills Kit (ASK), you can create tailored user experiences that cater specifically to your audience.
### 2. Implementing Machine Learning
Integrate machine learning models to improve interactions and personalize user experiences. For example, you could use AWS services like Amazon SageMaker to analyze user responses and provide tailored content based on their preferences.
### 3. Creating Multi-Language Skills
Consider developing multi-language skills to reach a broader audience. Alexa supports numerous languages, allowing you to create skills that cater to users in different regions.
### 4. Leveraging Voice User Interface (VUI) Design Principles
Good VUI design is crucial for creating a seamless user experience. Study VUI design principles to understand how to structure conversations, reduce user friction, and ensure that your skill is intuitive and user-friendly.
## Conclusion
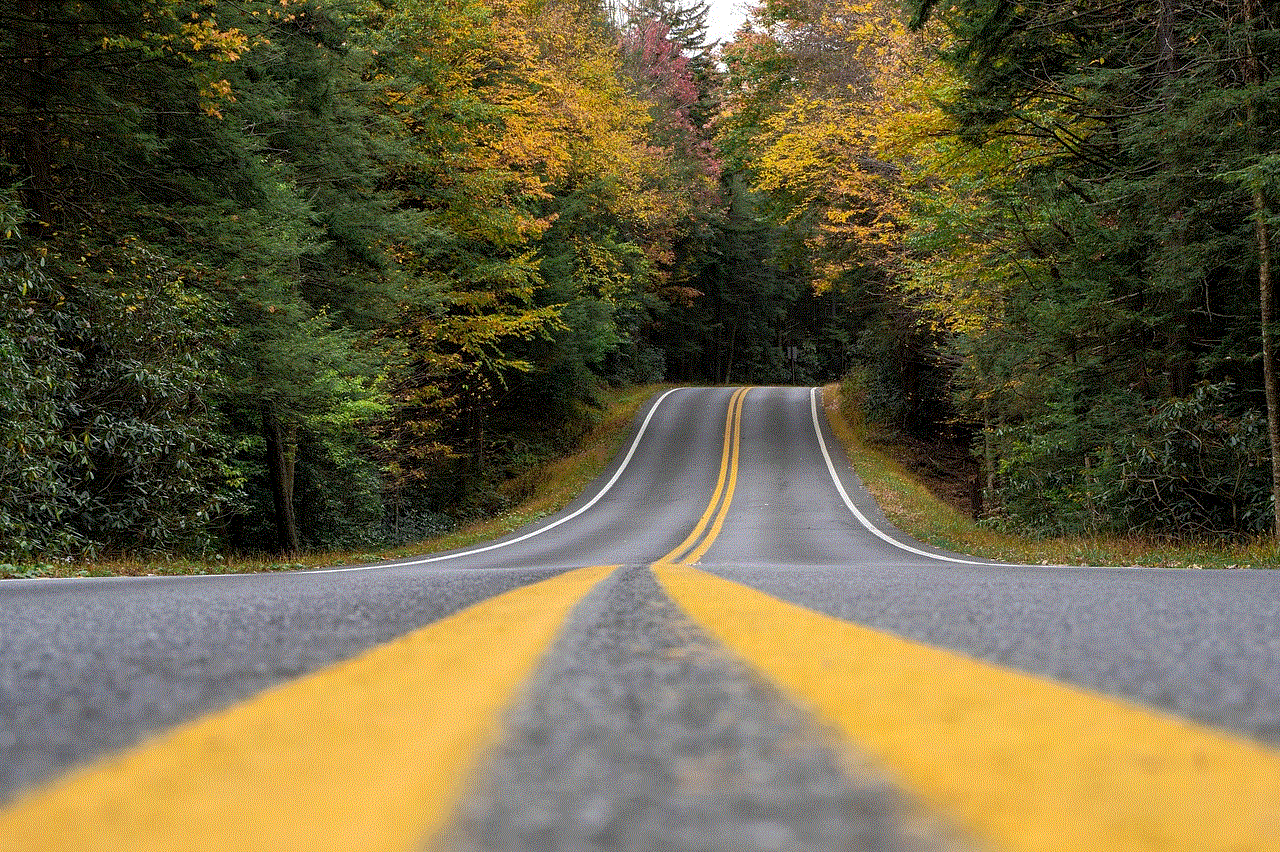
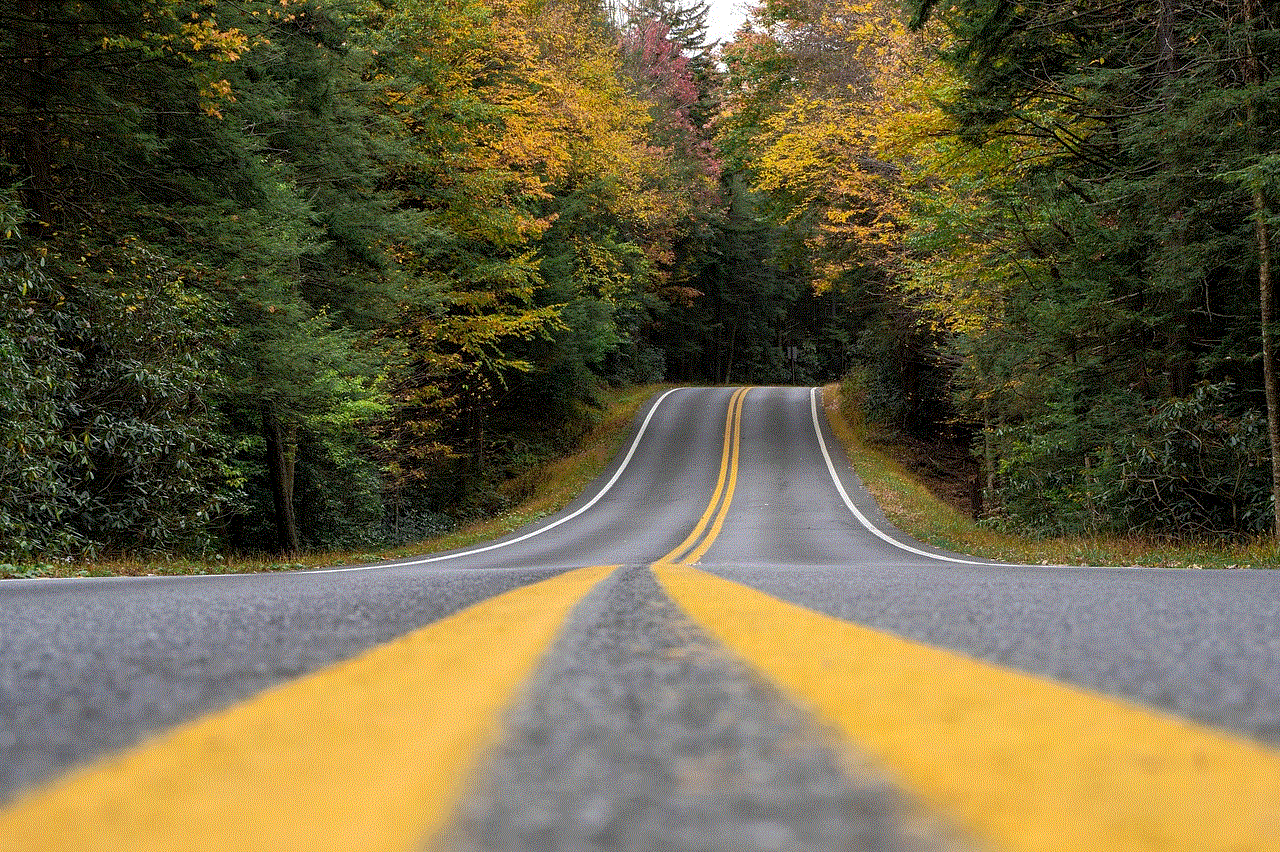
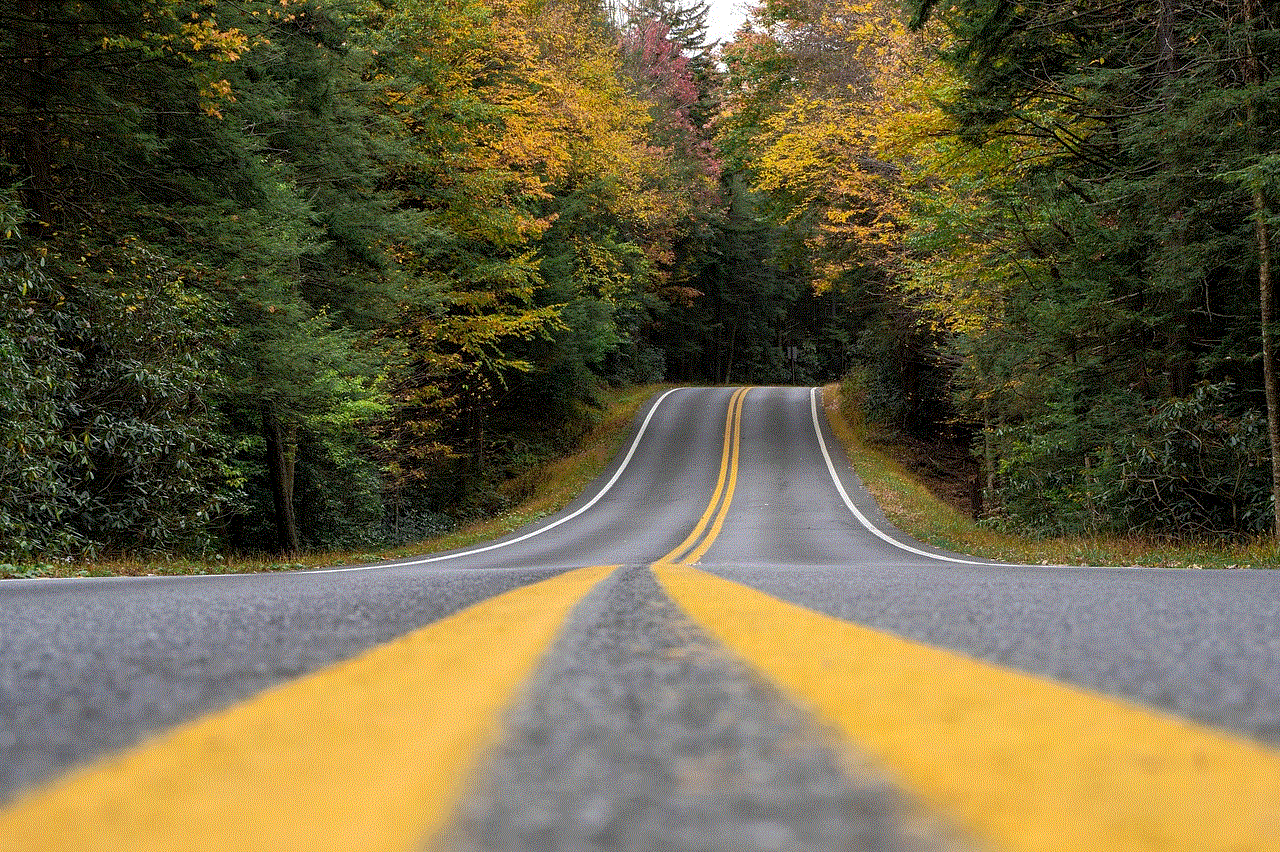
Programming Alexa is an exciting venture that opens up a world of possibilities for developers and enthusiasts alike. By understanding the capabilities of Alexa, setting up your development environment, and following the steps outlined in this guide, you can create engaging and functional Alexa skills. Whether you’re building a simple “Hello World” skill or a complex application that integrates with external APIs, the potential for innovation is limitless.
As you continue to develop your skills, remember to embrace a mindset of continuous learning. The technology landscape is ever-evolving, and staying updated with the latest advancements will ensure that your Alexa skills remain relevant and engaging. Happy coding!
0 Comments